In this quick post, I will show you how to build a Serverless function in Go to get the latest 9Gag Memes using OpenFaaS.
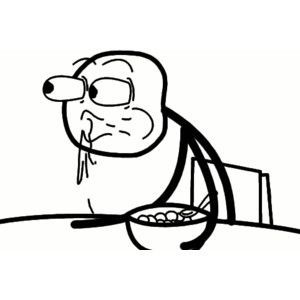
This tutorial assume that you have:
- faas-cli installed – The easiest way to install the faas-cli is through cURL:
1 | curl -sSL https://cli.openfaas.com | sudo sh |
- Swarm or Kubernetes environment configured – See Docs.
1 – Create a function
Create a handler.go file with the following content:
1 | package main |
The code is self-explanatory, it uses 9Gag Web Crawler to parse the website and fetch memes by their tag.
2 – Docker Image
I wrote a simple Dockerfile using the Multi-stage builds technique to reduce the image size down:
1 | FROM golang:1.9.1 AS builder |
3 – Configuration file
1 | provider: |
Note: If pushing to a remote registry change the name from mlabouardy to your own Hub account.
4 – Build
Issue the following command:
1 | faas-cli build -f ./stack.yml |
5 – Deploy
1 | faas-cli push -f ./stack.yml |
6 – Tests
Once deployed, you can invoke the function via:
cURL:
1 | curl http://localhost:8080/function/memes-9gag -d "GoT" |
FaaS CLI:
1 | echo "GoT" | faas-cli invoke memes-9gag |
UI:
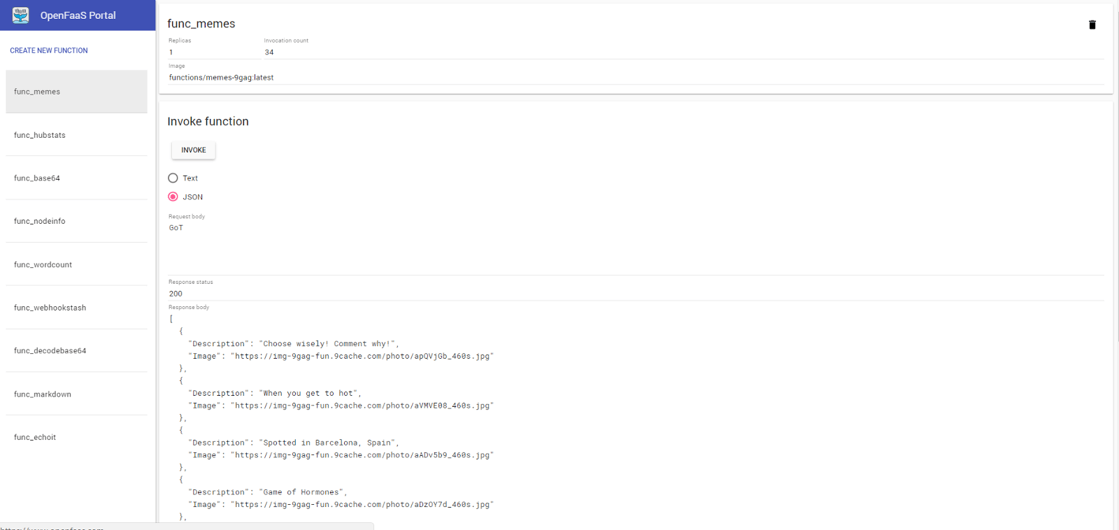
Note: all code used in this demo, is available on my GitHub 😍
Drop your comments, feedback, or suggestions below — or connect with me directly on Twitter @mlabouardy.